14 Mar Configuration Database
UVM configuration database, 즉, uvm_config_db
는 testbench component 간 data를 주고 받을 때 사용되는 interface 방식 중 한 가지다. 일반적으로 가장 많이 사용되는 method인 set
과 get
의 동작과 prototype은 다음과 같다.
- set – “cntxt 내 inst_name에 위치한 field_name이라는 이름의 data를 value의 값으로 set 해!”
- get – “cntxt 내 inst_name에 위치한 field_name이라는 이름의 data를 value에 get 해!”
static function void set(uvm_component cntxt, string inst_name, string field_name, T value)
static function bit get(uvm_component cntxt, string inst_name, string field_name, inout T value)
uvm_config_db
에 의해 set
된 database는 look-up table 형태로 관리되어 이를 기반으로 get
하게 된다. 이해를 돕기 위해 아래 그림과 code를 참고하자. Test에서 env에 2개, agent에 1개의 data를 set
하고 env와 agent에서 각각 get
하는 process를 나타낸다. 참고로 아래 simulation log는 Command Line Processors for Debugging 페이지에서 소개한 +UVM_CONFIG_DB_TRACE
옵션을 적용하여 출력한 결과로, configuration process에 대한 추가적인 정보를 확인하고자 할 때 도움이 된다.
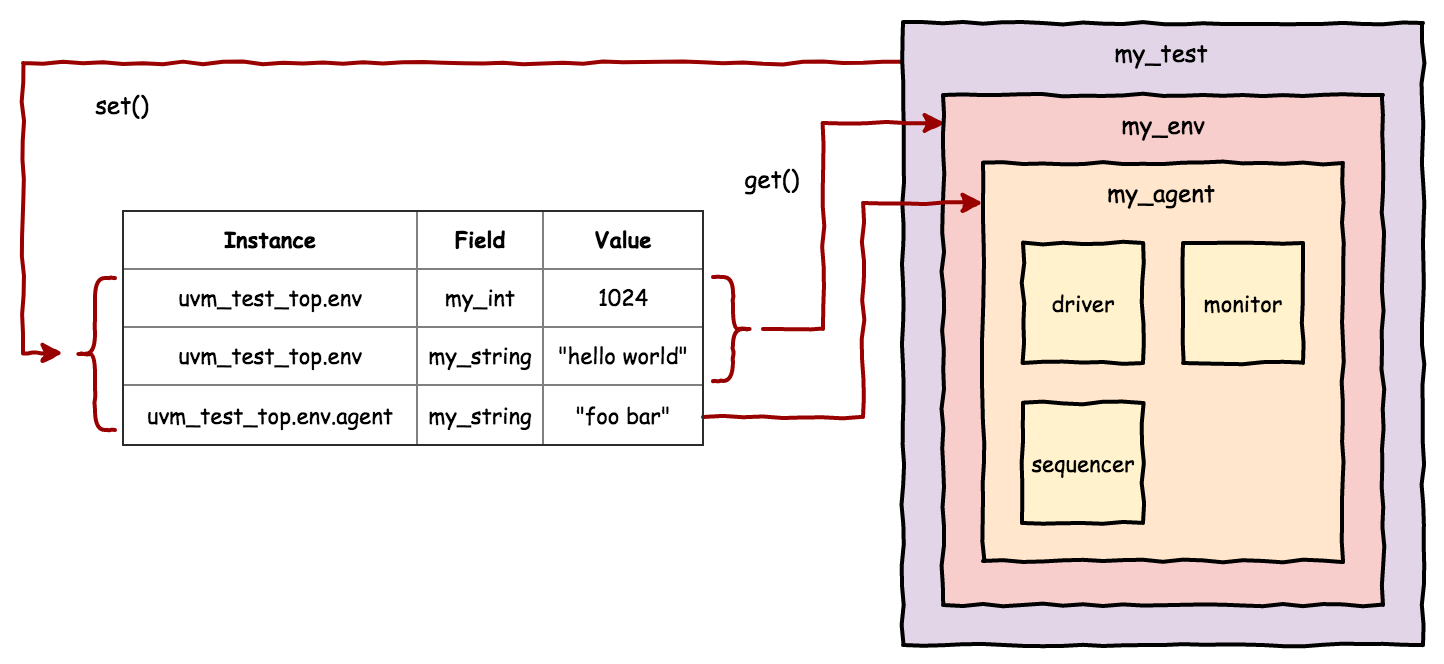
class my_test extends uvm_test; // ... function void build_phase(uvm_phase phase); // ... // set configuration database of type int on env uvm_config_db#(int)::set(this, "env", "my_int", 1024); // set configuration database of type string on env uvm_config_db#(string)::set(this, "env", "my_string", "hello world"); // set configuration database of type string on agent uvm_config_db#(string)::set(this, "env.agent", "my_string", "foo bar"); endfunction: build_phase endclass: my_test
class my_env extends uvm_env; // ... function void build_phase(uvm_phase phase); // ... // get configuration database of type int from env if (!uvm_config_db#(int)::get(this, "", "my_int", my_int)) begin my_int = -1; end // get configuration database of type string from env if (!uvm_config_db#(string)::get(this, "", "my_string", my_string)) begin my_string = "undefined"; end `uvm_info(get_type_name(), $sformatf("my_int = %1d", my_int), UVM_MEDIUM) `uvm_info(get_type_name(), $sformatf("my_string = %s", my_string), UVM_MEDIUM) endfunction: build_phase endclass: my_env
class my_agent extends uvm_agent; // ... function void build_phase(uvm_phase phase); // ... // get configuration database of type string from agent if (!uvm_config_db#(string)::get(this, "", "my_string", my_string)) begin my_string = "undefined"; end `uvm_info(get_type_name(), $sformatf("my_string = %s", my_string), UVM_MEDIUM) endfunction: build_phase endclass: my_agent
UVM_INFO @ 0: reporter [CFGDB/SET] Configuration 'uvm_test_top.env.my_int' (type int) set by uvm_test_top = (int) 1024 UVM_INFO @ 0: reporter [CFGDB/SET] Configuration 'uvm_test_top.env.my_string' (type string) set by uvm_test_top = (string) "hello world" UVM_INFO @ 0: reporter [CFGDB/SET] Configuration 'uvm_test_top.env.agent.my_string' (type string) set by uvm_test_top = (string) "foo bar" UVM_INFO @ 0: reporter [CFGDB/GET] Configuration 'uvm_test_top.env.my_int' (type int) read by uvm_test_top.env = (int) 1024 UVM_INFO @ 0: reporter [CFGDB/GET] Configuration 'uvm_test_top.env.my_string' (type string) read by uvm_test_top.env = (string) "hello world" UVM_INFO @ 0: uvm_test_top.env [env] my_int = 1024 UVM_INFO @ 0: uvm_test_top.env [env] my_string = hello world UVM_INFO @ 0: reporter [CFGDB/GET] Configuration 'uvm_test_top.env.agent.my_string' (type string) read by uvm_test_top.env.agent = (string) "foo bar" UVM_INFO @ 0: uvm_test_top.env.agent [agent] my_string = foo bar
위에서 보는 것과 같이 set
할 때는 cntxt
를 통해 database를 사용할 instance의 parent hierarchical path를 지정하고, inst_name
을 통해 해당 instance의 name을 지정한다. 결국 cntxt
와 inst_name
이 함께 하나의 instance를 가리킨다고 보면 된다. 문법상으로는 instance 지정 시 임의의 instance를 지정할 수 있고, wildcard를 이용하여 여러 instance에, 심지어는 전역에 set
할 수도 있지만, 의도치 않은 오동작을 방지하기 위하여 실제로 database를 사용할 instance를 가리키도록 coding 하는 것이 좋다. 특히 전역에 database를 set
하게 되면, database의 크기가 큰 경우 performance drop으로 이어질 수 있다. 마찬가지로 get
할 때도 database를 가져올 대상 instance가 get
을 call 하는 instance와 일치하는 것이 좋다.
또한 get
은 성공 여부에 따라 0 또는 1의 값을 반환하게 되는데, 이 반환값을 확인하여 실패 시 적절한 조치를 취하도록 coding 하는 것이 권장된다. 예를 들어 virtual interface를 get
하는 것과 같이 실패가 critical 한 경우 `uvm_fatal
을 이용하여 simulation을 종료시키고, 그렇지 않은 경우 의미 있는 default 값을 assign 하도록 한다.
// not recommended uvm_config_db#(uvm_bitstream_t)::get(this, "", "is_active", is_active); void'(uvm_config_db#(virtual my_if)::get(this, "", "my_vif", my_vif));
// recommended if (!uvm_config_db#(uvm_bitstream_t)::get(this, "", "is_active", is_active)) begin is_active = UVM_ACTIVE; end if (!uvm_config_db#(virtual my_if )::get(this, "", "my_vif", my_vif)) begin `uvm_fatal(get_name(), "virtual interface not set") end
마지막으로 uvm_config_db
와 유사한 uvm_resource_db
에 대한 설명은 Resource Database 페이지를 참고하되, 가급적 uvm_resource_db
대신 uvm_config_db
를 사용하는 것이 좋다.
References
- https://www.vmmcentral.com/uvm_vmm_ik/files3/base/uvm_config_db-svh.html
- https://www.synopsys.com/content/dam/synopsys/services/whitepapers/hierarchical-testbench-configuration-using-uvm.pdf

지나가는사람
Posted at 10:52h, 25 November좋은 글 감사합니다, 이해가 잘되네요 쉽게 설명하는 능력이 있으신거 같습니다ㅎㅎ
IKS
Posted at 13:13h, 25 November좋은 말씀 감사합니다. ^^
समलैंगिक अश्लील
Posted at 16:08h, 30 Marchमुष्टिप्रहार अश्लील qqyyooppxx.0fevtG9n5Ny
किन्नर अश्लीलता के बा
Posted at 00:14h, 31 Marchकिशोर अश्लीलता के बा hjkvbasdfzxzz.CVcs5j1UOOk
श्वेत-श्याम अश्लीलता
Posted at 19:32h, 01 Aprilतांडव अश्लील txechdyzxca.UTr3tjO7lCl
रूसी अश्लील साहित्य
Posted at 02:56h, 03 Aprilबीडीएसएम पोर्न है hkyonet.FjJm2cbn316
ladesbet ਖਿਡੌਣੇ ਪੋਰਨ ਹਨ
Posted at 13:59h, 05 Aprilladesbet ਵੱਡੇ ਗਧੇ ਪੋਰਨ ladesinemi.TnooLfhkLFM
ladesbet ウェブカメラ
Posted at 15:40h, 05 Aprilladesbet 異人種間のポルノ ladestinemi.f5lH8Qewel3